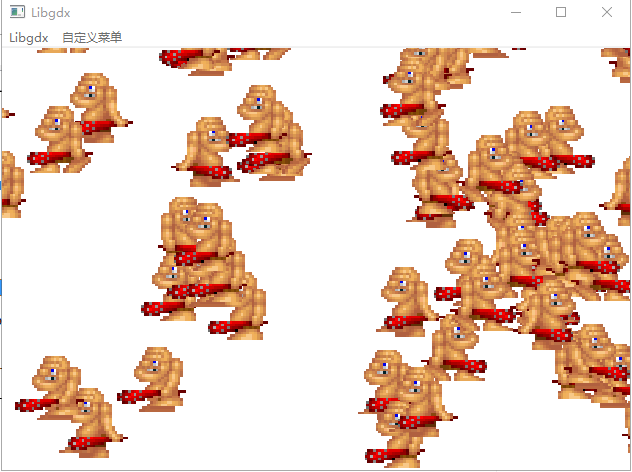
<?xml version="1.0" encoding="utf-8"?>
<Screen name="AnimationTest" descriptors="xworker.libgdx.Screen">
<actions>
<GroovyAction name="render">
<code><![CDATA[import com.badlogic.gdx.Gdx;
batch.begin();
for (int i = 0; i < cavemen.length; i++) {
def caveman = cavemen[i];
def frame = caveman.headsLeft ? leftWalk.getKeyFrame(caveman.stateTime, true) : rightWalk.getKeyFrame(
caveman.stateTime, true);
batch.draw(frame, caveman.pos.x, caveman.pos.y);
}
batch.end();
for (int i = 0; i < cavemen.length; i++) {
cavemen[i].update(Gdx.graphics.getDeltaTime());
}
fpsLog.log();]]></code>
</GroovyAction>
</actions>
<Resources>
<FileResource name="texture" type="texture" file="\data\walkanim.png"></FileResource>
<SpriteBatch name="batch" constructor="default"></SpriteBatch>
<FPSLogger name="fpsLog" _xmeta_id_="log"></FPSLogger>
</Resources>
<Code name="init">
<code><![CDATA[import com.badlogic.gdx.Gdx;
import com.badlogic.gdx.graphics.FPSLogger;
import com.badlogic.gdx.graphics.GL20;
import com.badlogic.gdx.graphics.Texture;
import com.badlogic.gdx.graphics.g2d.Animation;
import com.badlogic.gdx.graphics.g2d.SpriteBatch;
import com.badlogic.gdx.graphics.g2d.TextureRegion;
import com.badlogic.gdx.math.Vector2;
TextureRegion[] leftWalkFrames = TextureRegion.split(texture, 64, 64)[0];
TextureRegion[] rightWalkFrames = new TextureRegion[leftWalkFrames.length];
for (int i = 0; i < rightWalkFrames.length; i++) {
TextureRegion frame = new TextureRegion(leftWalkFrames[i]);
frame.flip(true, false);
rightWalkFrames[i] = frame;
}
leftWalk = new Animation(0.25f, leftWalkFrames);
rightWalk = new Animation(0.25f, rightWalkFrames);
cavemen = new Caveman[100];
for (int i = 0; i < 100; i++) {
cavemen[i] = new Caveman((float)Math.random() * Gdx.graphics.getWidth(),
(float)Math.random() * Gdx.graphics.getHeight(), Math.random() > 0.5 ? true : false);
}
class Caveman{
static final float VELOCITY = 20;
public final Vector2 pos;
public final boolean headsLeft;
public float stateTime;
public Caveman (float x, float y, boolean headsLeft) {
pos = new Vector2().set(x, y);
this.headsLeft = headsLeft;
this.stateTime = (float)Math.random();
}
public void update (float deltaTime) {
stateTime += deltaTime;
pos.x = pos.x + (headsLeft ? -VELOCITY * deltaTime : VELOCITY * deltaTime);
if (pos.x < -64) pos.x = Gdx.graphics.getWidth();
if (pos.x > Gdx.graphics.getWidth() + 64) pos.x = -64;
}
}]]></code>
</Code>
</Screen>